Blog
May 22, 2024
How to Use JMeter for Selenium WebDriver Testing
Performance Testing,
Open Source Automation
While JMeter is not a browser, it can look like a browser (or multiple browsers). Yet it does not perform every action supported by browsers — such as executing Javascript found in HTML pages or rendering HTML pages as a browser does.
So how can you marry JMeter and Selenium for WebDriver testing?
Although it is possible to configure JMeter to behave more like a real browser and ensure that it produces the same network footprint as the real user using the real browser does, there are certain areas which cannot be tested by JMeter — in particular, obtaining front-end performance metrics.
Of course it can be done using a browser automation framework like Selenium separately, but having both backend metrics from JMeter and frontend metrics from the real browser driven by Selenium would be really convenient; results will be in the same format and at the same place.
Fortunately, due to the modular architecture of JMeter, it can be extended by plugins. One of these existing plugins is WebDriver Sampler. This plugin provides a JMeter integration with Selenium so you can kick off a real browser from your JMeter test and measure metrics such as page load time, script execution time, and page rendering time.
This blog will discuss Selenium WebDriver and its limitations, how to install it, and how to overcome those limitations by using JMeter for WebDriver testing.
Selenium WebDriver Limitations
It is not recommended to use WebDriver Sampler for performance testing. There are two main reasons for that:
You will not get good results. With JMeter’s HTTP Request sampler you clearly see the relationship between concurrent requests and metrics/KPIs like response time, throughput, number of errors. With the WebDriver Sampler, you can only record timestamps and duration of larger chunks like “user opens the page in 2 seconds” but you don’t know how many requests were executed by the browser, what was the slowest one, etc.
- Browsers are very resource intensive. Even in headless mode. Firefox browser requires 1 CPU core at 1GHz and 2 GB of RAM per instance and this is the minimum. So you can run only a dozen of browsers on a modern mid-range laptop and with JMeter’s HTTP Request samplers you can get hundreds (if not thousands) virtual users simulated.
Therefore, the WebDriver Sampler is enriching your test results with the frontend metrics and not substituting HTTP Request samplers with real browsers.
Back to topInstalling JMeter for Selenium WebDriver Testing
It is recommended to install JMeter Plugins and keeping them up to date is via JMeter Plugins Manager.
To start the process of installing JMeter for Selenium WebDriver testing, open the JMeter Plugins Manager, look for the Selenium/WebDriver Support plugin, tick the box, and click “Apply Changes and Restart JMeter”:
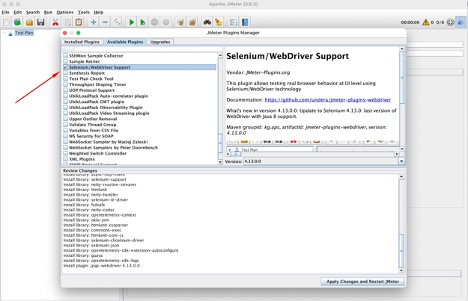
And after the JMeter Plugins Manager downloads, the WebDriver Sampler plugin and its dependencies JMeter will be restarted. After they restart, you will see WebDriver Sampler in the list of available Samplers:
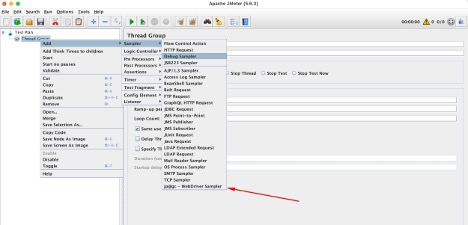
Getting Started With WebDriver Testing
In order to start using WebDriver Sampler you need to add a relevant Configuration Element first. For example:
- Chrome Driver Config - allows configuring Chromium based browsers.
- Firefox Driver Config - allows configuring Firefox browser.
- Remote Driver Config - allows using Selenium Grid from JMeter.
You can specify the relevant WebDriver binary pass command-line arguments, override Desired Capabilities, and so on.
The absolute minimum you need to provide is the path to WebDriver binary:
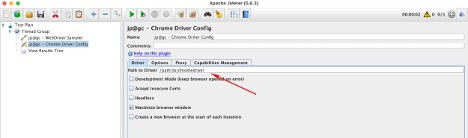
You can also provide location to the browser, configure proxy for the browser, and decide whether you want to keep the browser window open when the test ends or error occurs.
The Capabilities Management tab allows setting and overriding WebDriver Capabilities. For example, here is how you can configure your browser to accept non-secure connections (i.e. don’t show warning regarding self-signed certificate):
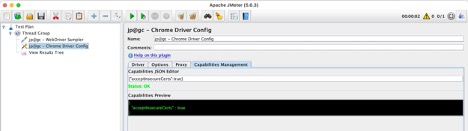
Writing JMeter Test Scripts for WebDriver Testing
Choose Your Language
The WebDriver Sampler itself is the place where you can put the code that will control the real browser, open pages, look for elements, perform clicks, type text, or do whatever you need to implement your frontend performance test scenario.
The recommended language for JMeter scripting is Groovy; you can find a lot of examples of code snippets in Java in the Selenium documentation and, in the majority of cases, valid Java code will be valid Groovy code.
WebDriver Sampler documentation and some legacy tutorials use JavaScript. It can still be used, but know that in Java 15+ there is no built-in JavaScript support and you will either need to get it back somehow (one option is via Mozilla Rhino) or switch to an alternative JVM like GraalVM.
Measure Execution Time
By default, the WebDriver Sampler reports the time of the code execution (excluding the time needed to start the browser). Everything between WDS.sampleResult.sampleStart() and WDS.sampleResult.sampleEnd() is reported as the Sampler execution time.
If you want to measure the execution time of smaller parts of your test code, there are two approaches:
- Use several WebDriver Samplers, one after the other:
First sampler code:
WDS.sampleResult.sampleStart()
WDS.browser.get('https://blazedemo.com')
WDS.sampleResult.sampleEnd()
Second sampler code:
import org.openqa.selenium.By
WDS.sampleResult.sampleStart()
WDS.browser.findElement(By.xpath('//input[@value="Find Flights"]')).click()
WDS.sampleResult.sampleEnd()
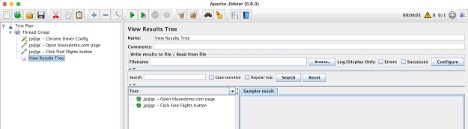
- Use SubResults — in this case you can create a tree-like structure similar to what you would use for embedded resources. You will need to set the following JMeter Property first:
webdriver.sampleresult_class=com.googlecode.jmeter.plugins.webdriver.sampler.SampleResultWithSubs
Once this is done, you can do the following:
import org.apache.jmeter.samplers.SampleResult
import org.openqa.selenium.By
WDS.sampleResult.sampleStart()
def subResult = new SampleResult()
subResult.setSampleLabel('Open system under test')
subResult.sampleStart()
WDS.browser.get('https://blazedemo.com')
subResult.sampleEnd()
subResult.setSuccessful(true)
WDS.sampleResult.addSubResult(subResult, false)
subResult = new SampleResult()
subResult.setSampleLabel('Click some button')
subResult.sampleStart()
WDS.browser.findElement(By.xpath('//input[@value="Find Flights"]')).click()
subResult.sampleEnd()
subResult.setSuccessful(true)
WDS.sampleResult.addSubResult(subResult, false)
WDS.sampleResult.sampleEnd()
And each “subResult” will be reported separately:
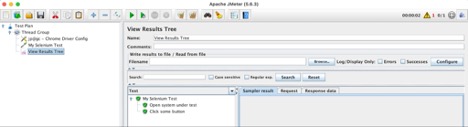
Conditionally Fail the WebDriver Sampler
As long as no exception occurs, the WebDriver Sampler will be marked as passed. If you want to fail the sampler if the expected condition is not met you will need to manually call a WDS.sampleResult.setSuccessful(false) function.
WDS.sampleResult.sampleStart()
WDS.browser.get('https://blazedemo.com')
def title = WDS.browser.getTitle()
if (title != 'BlazeMeter') {
WDS.sampleResult.setSuccessful(false)
WDS.sampleResult.setResponseCode('500')
WDS.sampleResult.setResponseMessage('Expected title to be "BlazeMeter", but got: "' + title + '"')
}
WDS.sampleResult.sampleEnd()
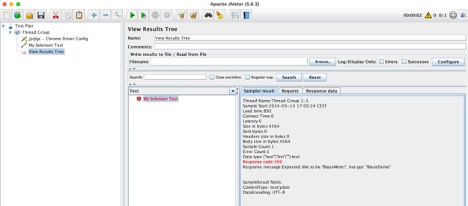
Work With JMeter API And Objects
The WebDriver Sampler exposes some predefined variables that can be used for passing data between Samplers, reading and writing JMeter Variables, getting information about runtime, and more.
For example:
- WDS.sampleResult stands for a SampleResult class instance.
- WDS.browser is a WebDriver instance.
- WDS.vars is for JMeterVariables.
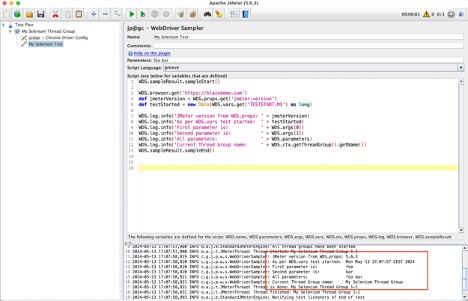
Bottom Line
JMeter is a powerful load testing tool that — thanks to its open-source nature — can be adapted for many different types of testing. However, when attempting to execute browser testing, it does come up a little short.
Using JMeter’s WebDriver Sampler plugin with your Selenium browser tests integrates the two platforms for seamless browser testing. Now that you know how to pair one with the other, you are well on your way to creating your own powerful and efficient browser tests using JMeter and Selenium.
Luckily, BlazeMeter amplifies the capabilities of both JMeter and Selenium with our industry-leading performance testing platform. See how we can take your browser tests to the next level — get started for FREE today!